Useful and common git commands for newbie programmer (feat. ssh-agent)
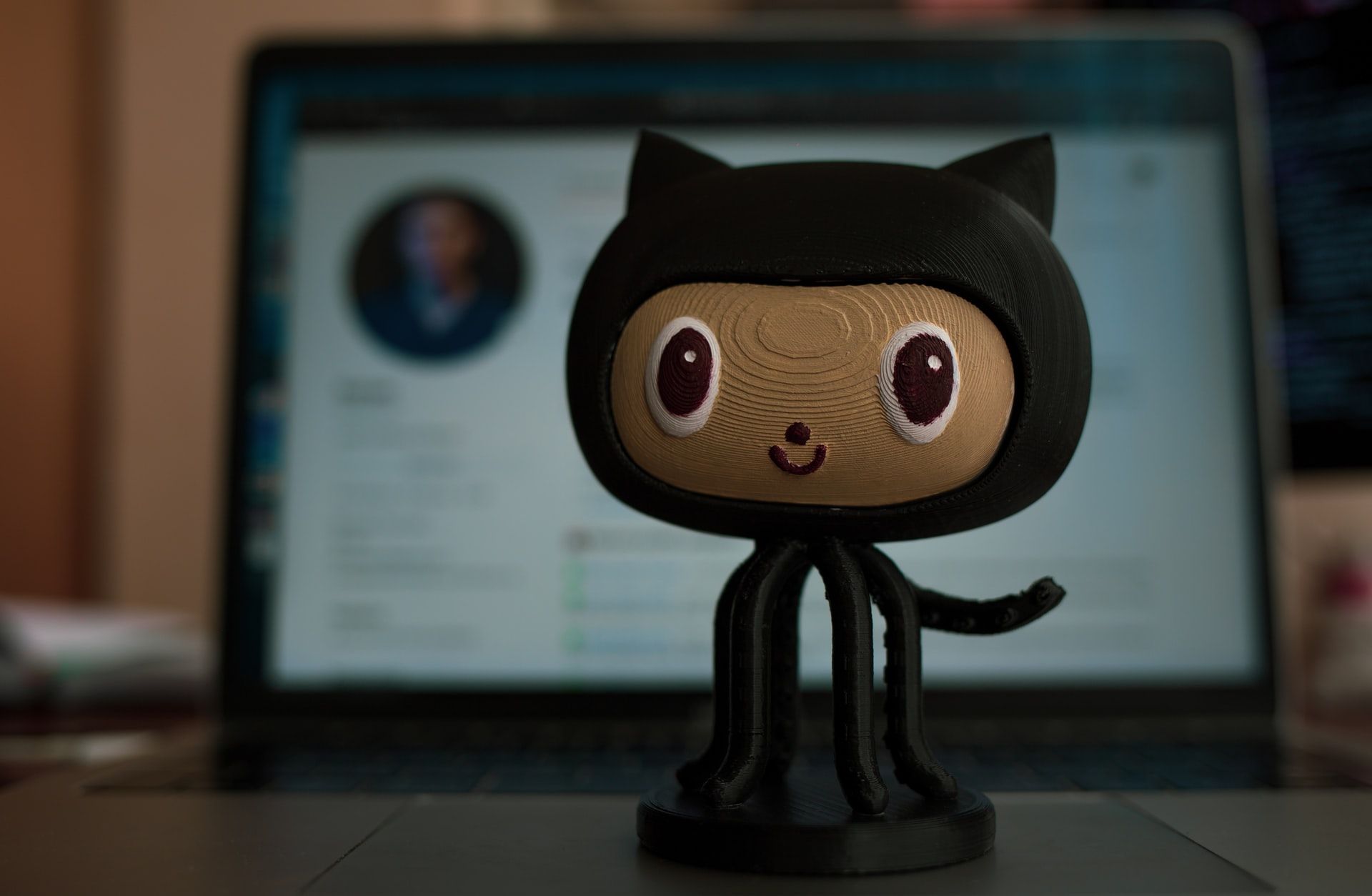
In this post, I assume your ssh key was already registered in your git hosting service such as Github, bitbucket, etc. If you don't know how to generate a new ssh key, you can search from your favourite search engine by typing a search keyword like github(or other alternative) generate ssh key
Just in case, I leave a page link for Github and Bitbucket in regards to this ssh key
Ok. Let's start!
Here are some useful commands that I am personally using often on my day-to-day works. First, to avoid any authentication issue with your git hosting service, start with ssh agent.
- Start ssh agent
eval $(ssh-agent -s)
- Add your private key
Please make sure to use the private key (file without having file extension such as ".pem" or ".ppk")
ssh-add yourpathtoprivatekey
For example, if your key is located under .ssh folder, then the command can be
ssh-add ~/.ssh/iamprivatekey
- Check list of keys
ssh-add -l
If your key is successfully added, then you should not see the "The agent has no identities." message
- Check your current branch
git branch
This will show the list of branch you have checked out. The current working branch will have the asterisk(*) in front of a branch name (e.g. * develop)
- Create new branch
If you need to make a code update on brand new branch, the use
git checkout -b new-branch-name
This will create the new branch and switch your current branch to the new branch.
- Switch your branch
If you or your team member already create a new branch from your git hosting service and you need to switch your branch, then use this command.
git fetch && git checkout new-branch
- Display the state of your working project directory
git status
This command will show you the list of files that have been modified, but show them into three different groups: staged, non-staged, untracked. Staged files are one will be committed. Non-staged files are simply files that are not staged for commit yet. Untracked files are the one not being tracked by Git.
- Add a file for commit
# Add your file to be staged
# Use git add yourfilepath
git add /src/main/java/com/test1/filetocommit.java
# If you want to add every file under test1 folder
git add /src/main/java/com/test1
Git status command after this will allow you to see the list of staged files in green text.
- Unstage a file
If you add a file by mistake and want to unstage, you can run
git restore --staged woops-not-this-file.java
- Discard your change of one file
git checkout yourfilepath/to/restore/back/to/original.java
- Commit your changes
Once you finish adding all files that you want to commit, run the following command.
git commit -m "Describe your changes in this double quotes, do not forget"
- Push your commit to your git repository
git push
- Merge one branch to the other branch
#Assume your working branch is called "feature-test"
git merge branch-name-that-you-want-to-merge
#For example, you want to merge to develop branch
git merge develop
However, merging branches wouldn't happen that often if you are working with other team members. In this case, a typical process would be as follows.
- Create a PR (Pull Request) from your Git hosting service
- Add your team member as a reviewer
- Team member review your PR and leave a feedback if needed
- Team member approve your PR
- Merge branch from your PR
So, in this post, I covered
- how to start ssh-agent and add the key to get proper authentication from your Git hosting service.
- how to use common git commands.
Hope this can be useful for someone. Happy coding!